Understanding the Unix Time Clock: A Comprehensive Guide
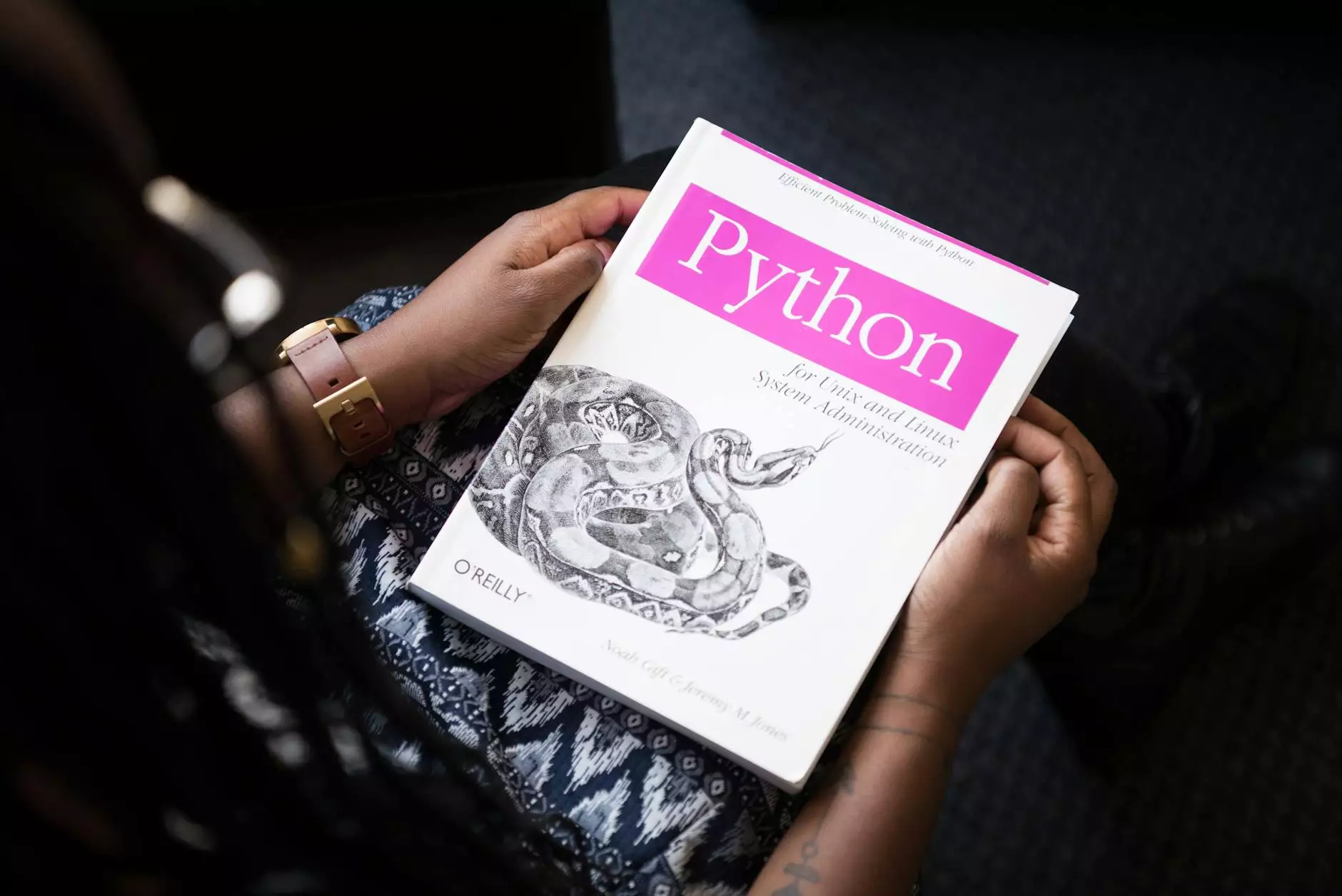
The Unix Time Clock is a crucial concept in the realms of programming, software development, and web design. As we delve deeper into its functionality, implications, and applications, we'll discover why the Unix time system has become a standard for timekeeping in computing.
What is Unix Time?
Unix time, also known as POSIX time or Epoch time, counts the number of seconds that have elapsed since the Unix epoch. This epoch began at 00:00:00 UTC on January 1, 1970, not counting leap seconds. The simplicity of this approach has made it an invaluable tool for developers.
The Importance of Unix Time Clock in Software Development
In software development, precision in timekeeping is critical. The Unix Time Clock provides a way to represent time as a single integer, which simplifies time arithmetic and comparisons.
Why Use Unix Time?
- Simplicity: It reduces a complex date-time format into a simple, continuous numeral.
- Cross-platform consistency: Since all systems use the same reference point (the epoch), it enables seamless time data transfer across various systems.
- Time zone independence: Unix time is universal, rendering it unaffected by time zones or daylight saving changes.
- Efficiency: Integer operations are faster than working with date and time objects, enhancing the performance of applications.
Implementing Unix Time in Applications
Using the Unix Time Clock in your applications can be straightforward. Most programming languages include libraries that facilitate Unix time conversions:
Example in Python
import time # Get the current Unix time current_time = int(time.time()) print("Current Unix Time:", current_time) # Convert Unix time to a readable format readable_time = time.strftime('%Y-%m-%d %H:%M:%S', time.localtime(current_time)) print("Readable Time:", readable_time)Example in JavaScript
// Get the current Unix time const currentTime = Math.floor(Date.now() / 1000); console.log("Current Unix Time:", currentTime); // Convert Unix time to a readable format const readableTime = new Date(currentTime * 1000).toLocaleString(); console.log("Readable Time:", readableTime);Best Practices for Using Unix Time in Web Design
When dealing with user interfaces and databases in web design, effective use of the Unix Time Clock can enhance user experience and data management:
Database Storage
- Store timestamps in Unix time: This ensures consistency and simplifies queries related to time.
- Convert for Display: Always convert Unix time to a human-readable format before displaying it to end-users.
User Interactions
Ensure that any time-based interactions, like countdowns or event schedules, are accurately computed using Unix time to avoid discrepancies that might confuse users.
Limitations of Unix Time
While the Unix Time Clock is incredibly useful, it does come with its limitations:
- Year 2038 Problem: Since Unix time is typically stored as a signed 32-bit integer, it will overflow on January 19, 2038. Programmers must consider this in long-term applications.
- Leap Seconds: Unix time does not account for leap seconds, which can introduce minor timing inaccuracies in highly precise applications.
Conclusion
The Unix Time Clock is a foundational element in modern computing, shaping how developers approach time-related programming tasks. Its efficiency, simplicity, and universality make it an indispensable tool in software development and web design.
Getting More from Your Unix Time Knowledge
Understanding the Unix Time Clock not only enhances your programming skills but also augments your ability to design robust applications. Consider how you can leverage this knowledge in your next project to achieve greater effectiveness and user satisfaction.
Further Resources
- Epoch Converter - A tool for converting Unix time to human-readable formats and vice versa.
- Wikipedia on Unix Time - In-depth information about Unix time, its history, and its implications.
- Tutorials Point - A resource for learning about Unix time in various programming languages.
By grasping the concept of the Unix Time Clock and its application across various facets of technology, you position yourself to excel in web design and software development. Embrace this knowledge, and you'll enhance your professional toolkit significantly.